In this program, user is asked to enter two integers and this program will add these two integers and display it.
In this program, user is asked to enter two integers. The two integers entered by user will be stored in variables num1 and num2 respectively. This is done using
Finally, the sum is displayed and program is terminated.
There are three variables used for performing this task but, you can perform this same task using 2 variables only. It can done by assiging the sum of

Source Code
/*C programming source code to add and display the sum of two integers entered by user */
#include <stdio.h>
int main( )
{
int num1, num2, sum;
printf("Enter two integers: ");
scanf("%d %d",&num1,&num2); /* Stores the two integer entered by user in variable num1 and num2 */
sum=num1+num2; /* Performs addition and stores it in variable sum */
printf("Sum: %d",sum); /* Displays sum */
return 0;
}
OutputEnter two integers: 12Explanation
11
Sum: 23
In this program, user is asked to enter two integers. The two integers entered by user will be stored in variables num1 and num2 respectively. This is done using
scanf( )
function. Then, + operator is used for adding variables num1 and num2 and this value is assigned to variable sum.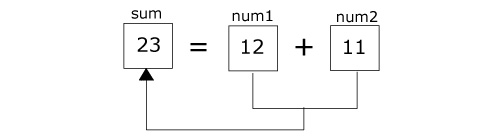
There are three variables used for performing this task but, you can perform this same task using 2 variables only. It can done by assiging the sum of
num1
and num2
to one of these variables as shown in figure below.
/* C programming source code to add and display the sum of two integers entered by user using two variables only. */
#include <stdio.h>
int main( )
{
int num1, num2, sum;
printf("Enter a two integers: ");
scanf("%d %d",&num1,&num2);
num1=num1+num2; /* Adds variables num1 and num2 and stores it in num1 */
printf("Sum: %d",num1); /* Displays value of num1 */
return 0;
}
This source code calculates the sum of two integers and displays it but, this program uses only two variables.
Post a Comment